Building a Telegram Bot with Node.js: A Step-by-Step Guide
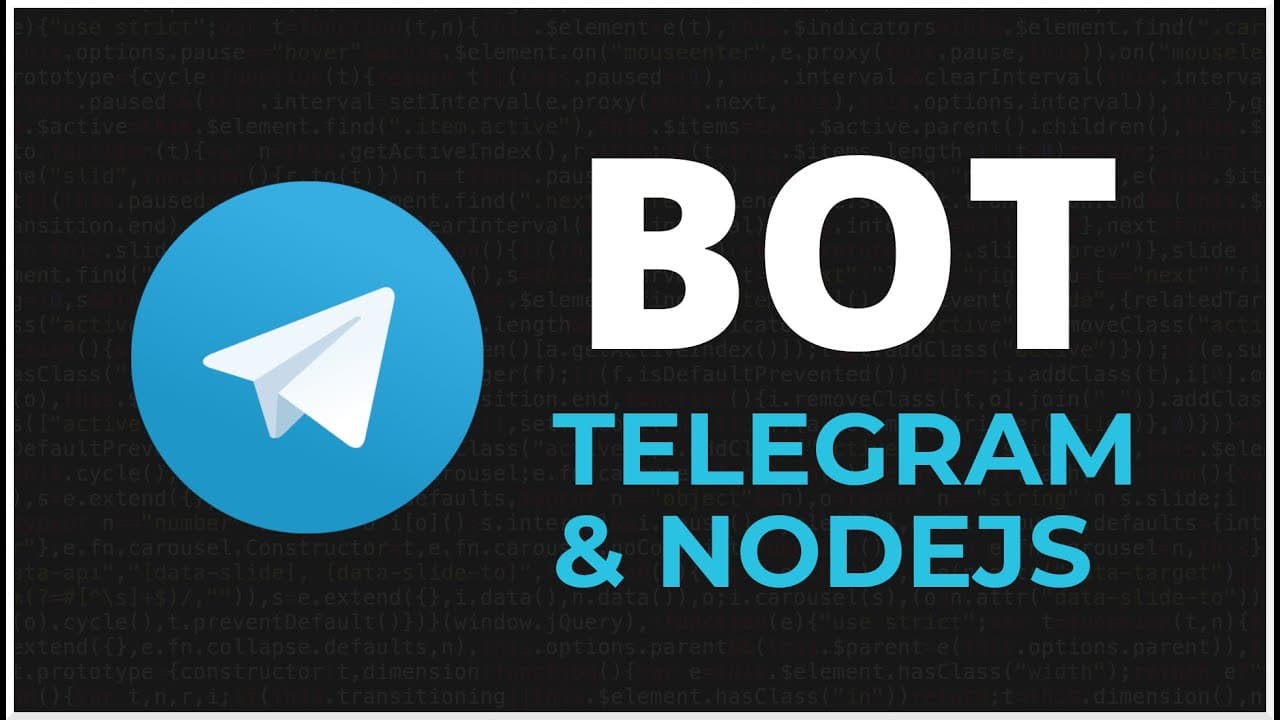
Telegram bots have become a powerful tool for automating tasks, providing information, and interacting with users. In this guide, we'll walk through the process of creating a Telegram bot using Node.js.
What is a Telegram Bot? A Telegram bot is an automated program that can interact with users on Telegram. Bots can perform various tasks such as sending messages, responding to commands, and integrating with external APIs.
Why Use Node.js for Telegram Bot Development? Node.js is an excellent choice for building Telegram bots due to its asynchronous nature and ease of integrating with external APIs. With Node.js, you can quickly set up a bot, handle requests, and interact with users in real-time.
Setting Up Your Environment Before you start building the bot, you'll need to set up your development environment.
Prerequisites Node.js installed on your system A Telegram account Step 1: Create a New Telegram Bot Open Telegram and search for the BotFather. Start a chat with BotFather and use the /newbot command to create a new bot. Follow the prompts to name your bot and choose a username. Once created, you'll receive a bot token. Keep this token safe as you'll need it to interact with the Telegram API. Step 2: Set Up a New Node.js Project mkdir telegram-bot cd telegram-bot npm init -y npm install node-fetch telegraf dotenv Here's a sample post about creating a Telegram bot using Node.js: yaml Copy code --- title: "Building a Telegram Bot with Node.js: A Step-by-Step Guide" date: "2024-09-03" author: "Faizan Hameed Tantray" tags: ["Node.js", "Telegram Bot", "Bot Development", "API Integration"] category: "Bot Development" description: "Learn how to create a Telegram bot using Node.js. This guide will walk you through the setup, coding, and deployment of your bot, enabling you to automate tasks and interact with users on Telegram." --- Telegram bots have become a powerful tool for automating tasks, providing information, and interacting with users. In this guide, we'll walk through the process of creating a Telegram bot using Node.js. What is a Telegram Bot? A Telegram bot is an automated program that can interact with users on Telegram. Bots can perform various tasks such as sending messages, responding to commands, and integrating with external APIs. Why Use Node.js for Telegram Bot Development? Node.js is an excellent choice for building Telegram bots due to its asynchronous nature and ease of integrating with external APIs. With Node.js, you can quickly set up a bot, handle requests, and interact with users in real-time. Setting Up Your Environment Before you start building the bot, you'll need to set up your development environment. Prerequisites Node.js installed on your system A Telegram account Step 1: Create a New Telegram Bot Open Telegram and search for the BotFather. Start a chat with BotFather and use the /newbot command to create a new bot. Follow the prompts to name your bot and choose a username. Once created, you'll receive a bot token. Keep this token safe as you'll need it to interact with the Telegram API. Step 2: Set Up a New Node.js Project bash Copy code mkdir telegram-bot cd telegram-bot npm init -y npm install node-fetch telegraf dotenv Step 3: Create a .env File In the root of your project, create a .env file to store your bot token securely. TELEGRAM_BOT_TOKEN=your_telegram_bot_token Step 4: Write the Bot Code Create an index.js file in the root directory and add the following code require('dotenv').config(); const { Telegraf } = require('telegraf'); const bot = new Telegraf(process.env.TELEGRAM_BOT_TOKEN); bot.start((ctx) => ctx.reply('Welcome to Faizan\'s Telegram Bot!')); bot.help((ctx) => ctx.reply('Send me a message and I\'ll echo it back to you!')); bot.on('text', (ctx) => { const userMessage = ctx.message.text; ctx.reply(`You said: ${userMessage}`); }); bot.launch(); console.log('Telegram bot is running...'); Step 5: Run Your Bot Start your bot with the following command: node index.js Your bot is now live and will respond to messages on Telegram. Deploying Your Telegram Bot Deploying to Heroku To keep your bot running 24/7, you can deploy it to a cloud platform like Heroku. Initialize a Git repository in your project folder: git init git add . git commit -m "Initial commit" Create a new Heroku app: heroku create set your bot token on Heroku: heroku config:set TELEGRAM_BOT_TOKEN=your_telegram_bot_token Push your code to Heroku: git push heroku main Your bot should now be live and running on Heroku. Conclusion Creating a Telegram bot using Node.js is straightforward and opens up a world of possibilities for automating tasks and interacting with users. This guide provided the basics, but there's much more you can do, such as integrating external APIs, handling more complex commands, and adding rich interactions like inline keyboards. Explore the Telegraf documentation to learn more about the capabilities of the library and enhance your bot's functionality. Happy coding!
Tags
Related Posts
About the Author
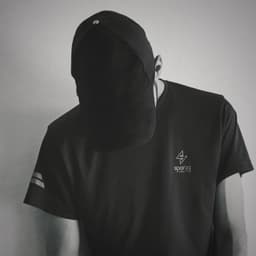
Faizan Hameed Tantray
A passionate writer and technologist exploring the intersections of code and creativity.