Mastering Full-Stack Development with Next.js and Node.js: A Complete Guide
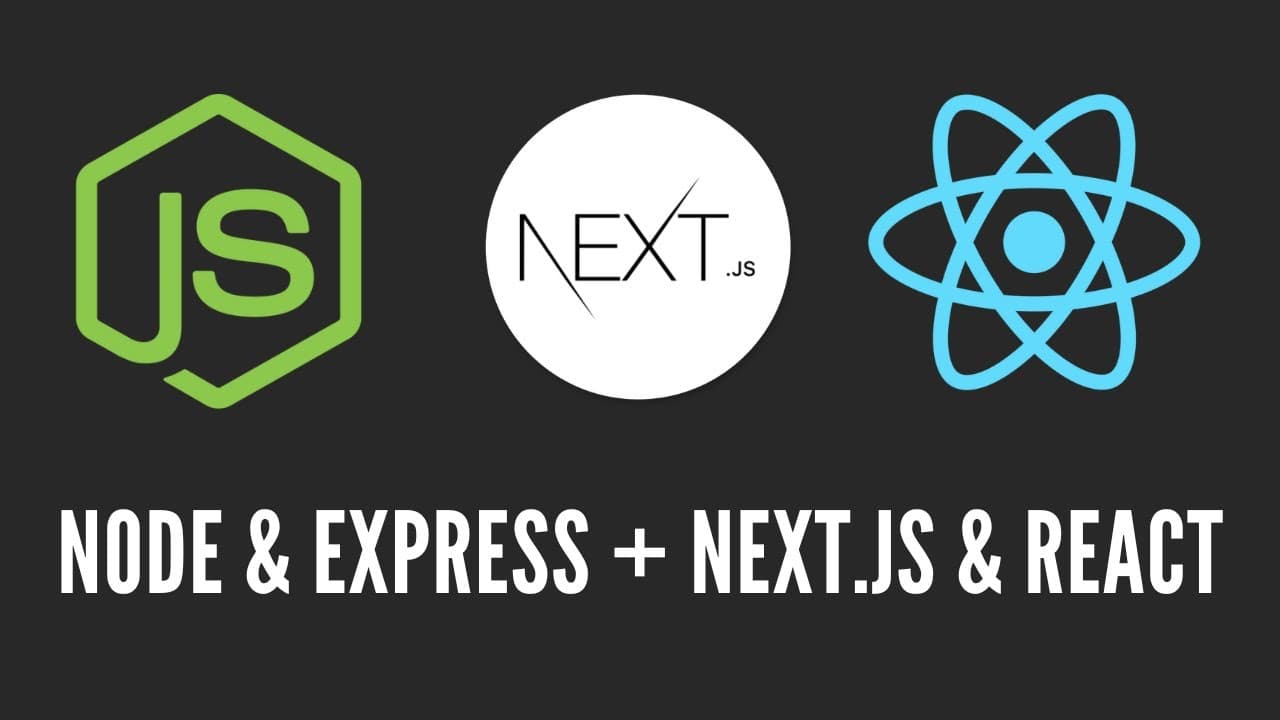
In the rapidly evolving landscape of web development, mastering full-stack development has become increasingly important. Being proficient in both frontend and backend technologies allows developers to create robust and scalable applications. In this guide, we'll explore how to use Next.js and Node.js to build a full-stack web application from scratch.
What is Full-Stack Development?
Full-stack development refers to the practice of working on both the frontend and backend of a web application. A full-stack developer is skilled in creating the user interface, as well as handling server-side logic, databases, and API integrations.
Why Next.js and Node.js?
Next.js is a powerful React framework that simplifies the process of building server-rendered applications and static websites. It offers features like automatic code splitting, optimized performance, and built-in routing.
Node.js, on the other hand, is a runtime environment that allows developers to run JavaScript on the server side. Its non-blocking I/O model makes it ideal for building scalable network applications.
Combining Next.js with Node.js gives you a versatile stack capable of handling the entire application lifecycle, from request handling to rendering the final HTML.
Setting Up Your Environment
Before we dive into building the application, let’s set up our development environment.
Create a new Next.js project:
npx create-next-app fullstack-app
cd fullstack-app
Set up the backend with Node.js:
Create a new directory for your backend:
mkdir backend
cd backend
npm init -y
npm install express mongoose cors dotenv
Configure your backend:
Create a file named server.js in the backend directory:
javascript
const express = require('express');
const mongoose = require('mongoose');
const cors = require('cors');
require('dotenv').config();
const app = express();
app.use(cors());
app.use(express.json());
mongoose.connect(process.env.MONGO_URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
}).then(() => {
console.log('Connected to MongoDB');
}).catch(err => console.log(err));
app.get('/', (req, res) => {
res.send('Hello from the backend!');
});
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
Create a .env file:
In the backend directory, create a .env file with the following content:
MONGO_URI=your_mongo_db_connection_string
Building the Application
Frontend: Connecting Next.js to the Backend
In the frontend, we’ll create a simple form that interacts with our Node.js backend. This will involve making API requests to fetch and submit data.
Update pages/index.js:
import { useState } from 'react';
export default function Home() {
const [data, setData] = useState('');
const fetchData = async () => {
const res = await fetch('http://localhost:5000');
const result = await res.text();
setData(result);
};
return (
<div className="container">
<h1 className="text-2xl">Welcome to Full-Stack Development</h1>
<button onClick={fetchData} className="mt-4 bg-blue-500 text-white p-2 rounded">
Fetch Data from Backend
</button>
{data && <p className="mt-4">{data}</p>}
</div>
);
}
Run the frontend and backend:
Start the Next.js frontend:
npm run dev
Start the Node.js backend:
node backend/server.js
You should now have a full-stack application where the frontend interacts with the backend.
Deploying the Application
Deploying with Vercel and Heroku
Deploy the frontend to Vercel: Follow the Vercel deployment guide for Next.js to deploy your frontend.
Deploy the backend to Heroku: Push your backend to Heroku using the Heroku CLI.
Connecting the Frontend and Backend in Production:
Update the API endpoints in your frontend to point to your Heroku app instead of localhost.
Conclusion
Building a full-stack application with Next.js and Node.js offers a comprehensive approach to web development. This guide covered the basic setup and interaction between the frontend and backend, but there's much more you can do. Explore integrating authentication, databases, and advanced state management to take your application to the next level.
By mastering these tools, you'll be well-equipped to build modern, scalable, and performant web applications. Happy coding!
Created with ❤ by Faizan Hameed
Tags
Related Posts
Mastering Dark Web Development: Building and Deploying a Hidden Service Website
2024-10-01
About the Author
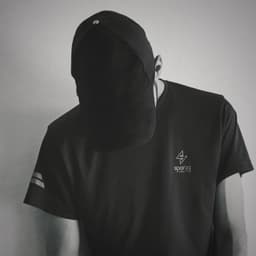
Faizan Hameed Tantray
A passionate writer and technologist exploring the intersections of code and creativity.